Introduction
In the vast ocean of software development, one encounters inevitable roadblocks known as exceptions. They are runtime errors that disrupt the smooth execution of programs, potentially leading to complete program failures or unpredictable behavior. The Java programming language provides a robust exception-handling mechanism to combat this challenge and maintain program stability.
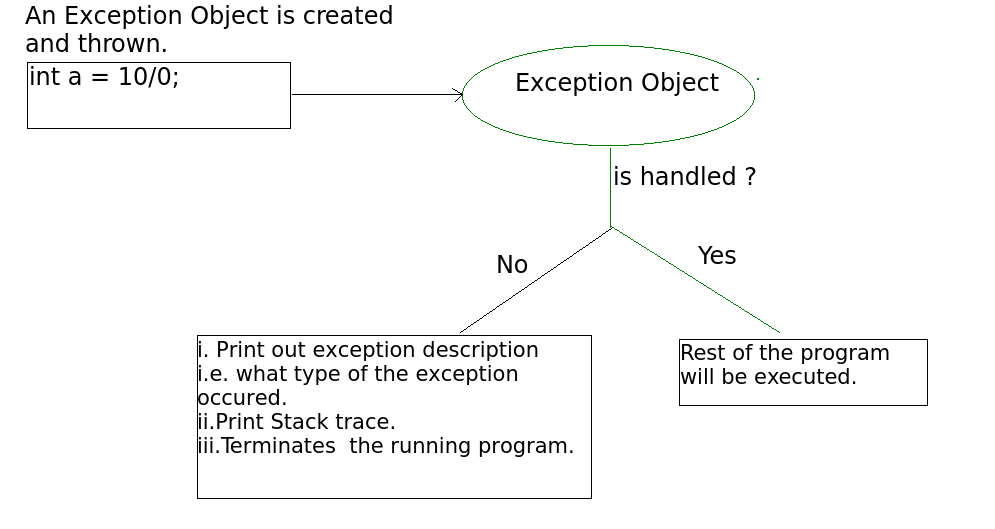
Image: nhanvietluanvan.com
Exception handling involves detecting and responding to exceptions in a controlled manner, allowing developers to gracefully recover from errors and maintain program flow. This article delves into the nuts and bolts of Java exception handling, exploring its fundamentals, best practices, and real-world applications, empowering you to write resilient and fault-tolerant code.
Understanding Exception Classes and Inheritance
At the core of Java’s exception-handling system lies a comprehensive hierarchy of exception classes that provide a structured classification of potential errors. The base class of all exceptions is the Exception class, from which all other exceptions inherit. This inheritance structure allows for the differentiation of specific error conditions and tailored handling.
Unchecked Exceptions: These exceptions, derived from the RuntimeException class, represent conditions that could not reasonably be anticipated or prevented at compile time. Examples include attempts to access array elements out of bounds or casting errors. Unchecked exceptions are not required to be declared or caught by the compiler, providing developers with flexibility in handling these errors.
Checked Exceptions: In contrast, checked exceptions, derived from the Throwable class, represent conditions that can be detected and handled during compilation. These exceptions reflect errors that are considered programming flaws, such as file input/output (I/O) errors or database connectivity issues. Checked exceptions must be explicitly declared in method signatures or caught in try-catch blocks, ensuring that potential errors are not silently ignored.
Catching Exceptions with try-catch Blocks
The try-catch block is the cornerstone of exception handling in Java. It provides a structured mechanism to capture and handle exceptions gracefully. The syntax is as follows:
try
// Code block where exception may occur
catch (ExceptionType1 e1)
// Code block to handle ExceptionType1
catch (ExceptionType2 e2)
// Code block to handle ExceptionType2
catch (...)
// Code block to handle all remaining exceptions
Each catch block corresponds to a specific ExceptionType, providing custom handling for different exception scenarios. If an exception occurs within the try block, execution will proceed to the corresponding catch block, allowing for appropriate error handling.
Exception Propagation and Rethrowing
There are situations where an exception cannot be handled within a particular scope. Exception propagation allows an exception to be passed up the call stack to be handled by a higher-level scope. This is useful when a method does not have the necessary context or logic to handle the exception.
Using the throws keyword in a method signature declares that the method may throw a particular exception. This propagates the exception to the calling method, which is then responsible for handling it. Rethrowing an exception is another way to pass it up the call stack, providing additional context or error handling at higher levels.
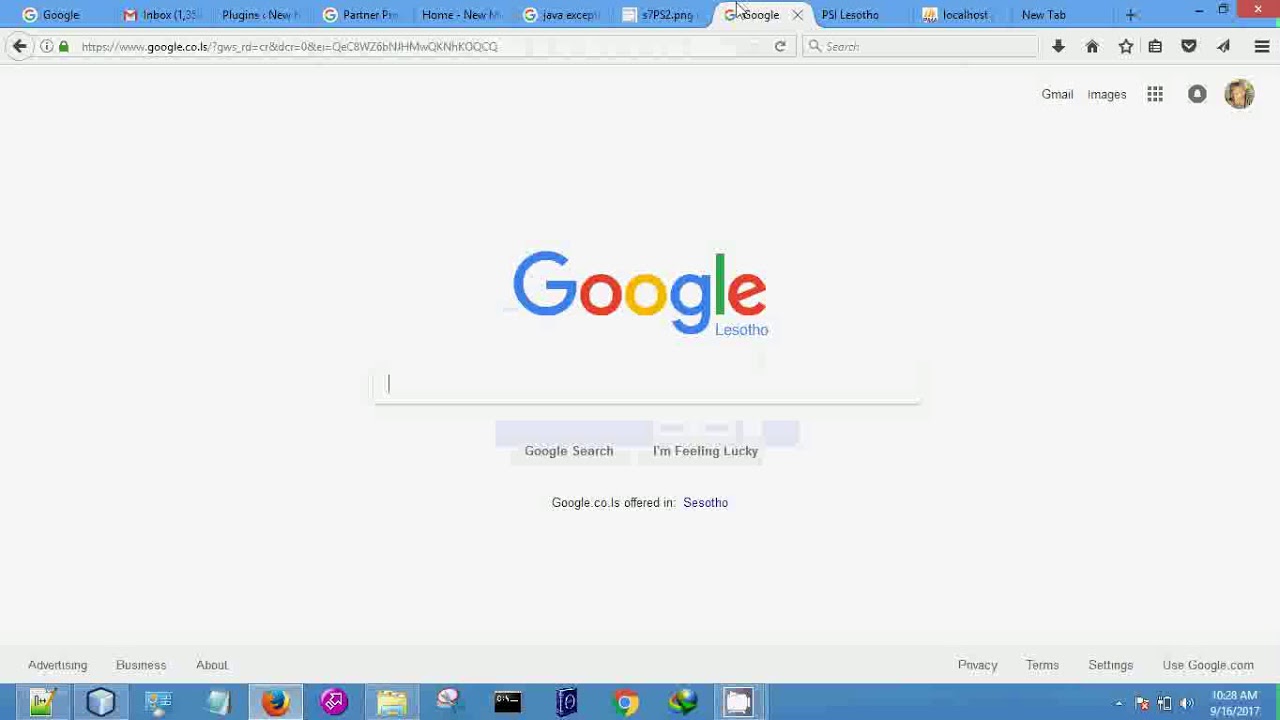
Image: www.youtube.com
Custom Exception Classes for Clear Error Reporting
Creating custom exception classes enhances the expressiveness and clarity of error reporting. Instead of relying on generic exception types, custom exceptions can provide specific information about the error condition, making it easier for developers to identify and resolve issues.
A custom exception class should provide a descriptive name and implement the Exception class. It can include additional fields and methods to capture contextual information, such as error codes, messages, and suggestions for resolution.
Best Practices for Effective Exception Handling
Adopting best practices for exception handling leads to robust and maintainable code. Here are some fundamental guidelines for handling exceptions effectively:
• Use checked exceptions sparingly: Throwing checked exceptions should be limited to situations where timely handling is crucial to prevent system failures or data corruption.
• Handle exceptions as close to the source as possible: Exceptions should be handled at the point where they occur, allowing for precise error handling and preventing unnecessary propagation.
• Log and notify: Logging exceptions provides valuable insights into potential problems, enabling early detection and resolution. In critical situations, notification systems can be used to alert responsible parties of urgent errors.
• Use exception hierarchies wisely: Leveraging Java’s exception hierarchy enables selective exception handling, tailoring recovery logic to specific error scenarios.
• Don’t ignore exceptions: Ignoring exceptions can lead to unexpected behavior and system failures. It’s essential to handle exceptions proactively to ensure program stability.
How To Fix A Java Exception Has Occurred
Conclusion
Java exception handling provides a powerful mechanism to handle runtime errors, ensuring program stability and resilience in the face of unexpected conditions. By understanding the mechanics of exception classes, utilizing try-catch blocks, and adhering to best practices, developers can write robust and reliable code that adapts seamlessly to changing conditions. Exception handling is an essential skill in the arsenal of every Java programmer, empowering them to navigate the complexities of software development and deliver exceptional software products.